Creating a Ribbon Add-in for Office Word in NetOffice Visual Studio Express 2008 without VSTO
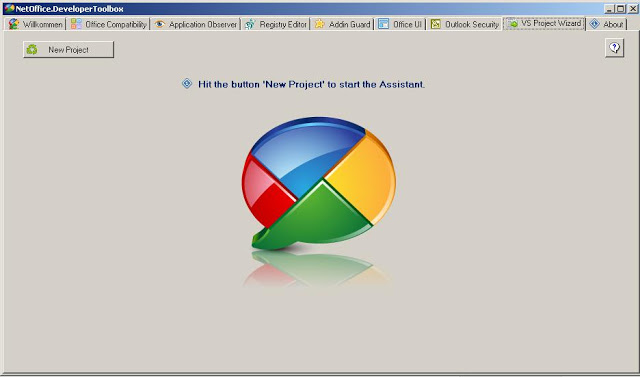
learnings of a young programmer
I will be using versions OpenCV 2.4.2 and Tesseract OCR 3.02.02.
Equation OCR Part 1: Using contours to extract characters in OpenCV
Equation OCR Part 2: Training characters with Tesseract OCR
Equation OCR Part 3: Equation OCR
Installing Tesseract: http://blog.ayoungprogrammer.com/2012/11/tutorial-installing-tesseract-ocr-30202.html/
OpenCV : http://opencv.org/
Tesseract OCR: http://code.google.com/p/tesseract-ocr/
I will be using versions OpenCV 2.4.2 and Tesseract OCR 3.02.02.
Equation OCR Part 1: Using contours to extract characters in OpenCV
Equation OCR Part 2: Training characters with Tesseract OCR
Equation OCR Part 3: Equation OCR
Installing Tesseract: http://blog.ayoungprogrammer.com/2012/11/tutorial-installing-tesseract-ocr-30202.html/
OpenCV : http://opencv.org/
Tesseract OCR: http://code.google.com/p/tesseract-ocr/
To start the training: (mat for math)
tesseract mat.arial.exp0.tif mat.arial.exp0 nobatch box.train
Now you will see that Tesseract has generated a file called mat.arial.exp0.tr. Don’t touch the file. Next we will have to tell Tesseract which possible characters we are using. This can be generated by running:
uncharset_extractor mat.arial.exp0.box
Create a new file called font_properties (no file type like the unicharset, I just copied the unicharset file and save it under a new name called font_properties). Do not use notepad as it will mess up formatting. Use something like WordPad. Inside font_properties type in:
arial 1 0 0 1 0
Next to start mftraining:
mftraining -F font_properties -U unicharset mat.arial.exp0.tr
Shape clustering:
shapeclustering -F font_properties -U unicharset mat.arial.exp0.tr
mftraining again for shapetables:
mftraining -F font_properties -U unicharset mat.arial.exp0.tr
cntraining for clustering:
Now we have to combine all these files into one file. Now rename all the following files:
inttemp -> mat.inttemp
shapetable -> mat.shapetable
normproto -> mat.normproto
pffmtable -> mat.pffmtable
unicharset -> mat.unicharset
To generate your new tess data file:
combine_tessdata mat.
The final generated file is mat.traineddata. Move this file into the tessdata folder in the Tesseract installation folder so that the Tesseract library can access it -> C:Program FilesTesseract-OCRtessdata
To test go into one of your test data folders like “1” and run tesseract with your language file:
tesseract 1.jpg output -l mat -psm 10
In the output file you should see the character “1”. Congratulations, you have just trained your first OCR language!
I will be using versions OpenCV 2.4.2 and Tesseract OCR 3.02.02.
Equation OCR Part 1: Using contours to extract characters in OpenCV
Equation OCR Part 2: Training characters with Tesseract OCR
Equation OCR Part 3: Equation OCR
Installing Tesseract: http://blog.ayoungprogrammer.com/2012/11/tutorial-installing-tesseract-ocr-30202.html/
OpenCV : http://opencv.org/
Tesseract OCR: http://code.google.com/p/tesseract-ocr/
Mat mask = Mat::zeros(image.size(), CV_8UC1);
drawContours(mask, contours_poly, i, Scalar(255), CV_FILLED);
Here are some sample equations you can use:
Source code is available here: http://pastebin.com/Q2x8kHmG
More updated tutorial: https://github.com/gulakov/tesseract-ocr-sample
1. Download and install the full windows version of Tesseract. This way you won’t have to extract all the different separate files.
http://code.google.com/p/tesseract-ocr/downloads/detail?name=tesseract-ocr-setup-3.02.02.exe
Leave the destination folder as the default (C:Program FilesTesseract-OCR)
Remember to check Tesseract Development files!
2. Open up Microsoft Visual Studio 2008 and go to Tools -> Options
Project solutions -> VC++ Directories -> Show directories for include files
Add:
C:Program FilesTesseract-OCRinclude
C:Program FilesTesseract-OCRincludetesseract
C:Program FilesTesseract-OCRincludeleptonica
3. Next click show directories for -> Library Files
Add:
C:Program FilesTesseract-OCRlib
4. Configure linker options for Tesseract
Right click your project in solution explorer and click properties
Configuration Properties -> Linker->Input ->Additional Dependencies
Add this in there:
libtesseract302.lib
libtesseract302d.lib
liblept168.lib
liblept168d.lib
**You will have to do this for every project
***I think you can do this with the property sheets but I don’t know how to set it up. Message me if you do!
5. Copy liblept168.dll, liblept168d.dll, libtesseract302.dll and libtesseract302.dll from C:Program FilesTesseract-OCR into your project folder (Optional)
If for some reason when you run your program and you get .dll missing add these files into your project folder.
6. Hello World!
To check if your project works create your main cpp file with this code:
#include <baseapi.h>
#include <allheaders.h>
#include <iostream>
using namespace std;
int main(void){
tesseract::TessBaseAPI api;
api.Init(“”, “eng”, tesseract::OEM_DEFAULT);
api.SetPageSegMode(static_cast<tesseract::PageSegMode>(7));
api.SetOutputName(“out”);
cout<<“File name:”;
char image[256];
cin>>image;
PIX *pixs = pixRead(image);
STRING text_out;
api.ProcessPages(image, NULL, 0, &text_out);
cout<<text_out.string();
}
Copy this image into your project folder: (Right click save file as)
Copy eng.traineddata from C:Program FilesTesseract-OCRtessdata into your project folder and it should output Hello World! The traineddata file will be used as the data file for reading the text.
More to come! I will be making a tutorial maybe next week on linking OpenCV with Tesseract and maybe also on how to train Tesseract.
The OpenCV library is an open source library which means it is free to use but it also means that the documentation is not the greatest. Trying to install OpenCV and getting a program to run took me 3 hours going through various tutorials and guides. The legitimate OpenCV guide to installing did not work for me as well as the other guides. I combined a couple of methods together to get it finally working after hours of frustration.
Anyways that was my spiel on computer vision. I have Microsoft Visual Studio 2008 Express installed on my computer on a Windows Vista (x86 machine). These are the steps I used to get OpenCV working on my computer:
1. Download the OpenCV Project
Download the file at: http://sourceforge.net/projects/opencvlibrary/files/opencv-win/2.4.2/OpenCV-2.4.2.exe/download and run the .exe. The application should have extracted a bunch of files into a folder called opencv.
2. Download CMake
Now download CMake which will be used to “build” the project: http://www.cmake.org/files/v2.8/cmake-2.8.9-win32-x86.exe.
3. Run the CMake GUI program.
Where is the source code: <your OpenCV folder>
Where to build: <your OpenCV folder/build>
4. Build the binaries for your OpenCV library
Click configure and select Visual Studio 9 2008.
Click Finish and it will output some information on your compiler.
5. Keep clicking generate until you get no more red text.
6. Configure in Visual Studio 2008 Express
Once you’re done that, open up Visual Studio 2008 Express. Tools -> options
In your “include files” add the following directories:
<your opencv folder>buildinclude
<your opencv folder>buildincludeopencv
In your “library files” add the following directories:
<your opencv folder>buildx86vc9lib
7. Configure .dlls to be used in system
Computer-> right click (properties) -> Advanced system settings
In user variables, go to path and click edit.
Add this line exactly as it is to the variable value. Key word: add. If you delete everything in there, you’re going to have a bad time.
;<your OpenCV folder>buildx86vc9bin>;<your openCV folderbuildcommontbbia32vc9
8. Create Hello World in OpenCV
Create a new Visual Studio 2008 Express projects. File-> New -> Project.
An empty win32 console project will be fine.
Create a new .cpp file and copy this hello world code into it:
#include <cv.h> #include <highgui.h> int main ( int argc, char **argv ) { cvNamedWindow( "My Window", 1 ); IplImage *img = cvCreateImage( cvSize( 640, 480 ), IPL_DEPTH_8U, 1 ); CvFont font; double hScale = 1.0; double vScale = 1.0; int lineWidth = 1; cvInitFont( &font, CV_FONT_HERSHEY_SIMPLEX | CV_FONT_ITALIC, hScale, vScale, 0, lineWidth ); cvPutText( img, "Hello World!", cvPoint( 200, 400 ), &font, cvScalar( 255, 255, 0 ) ); cvShowImage( "My Window", img ); cvWaitKey(); return 0; }
Before we can compile it, first we need to link the opencv libraries with our own project. This can be done by right clicking your project in visual studio and clicking properties.
Go to linker->input and add to additional dependencies:
opencv_core242d.lib
opencv_imgproc242d.lib
opencv_highgui242d.lib
opencv_ml242d.lib
opencv_video242d.lib
opencv_features2d242d.lib
opencv_calib3d242d.lib
opencv_objdetect242d.lib
opencv_contrib242d.lib
opencv_legacy242d.lib
opencv_flann242d.lib
You will need to do this for every project you make (I’m not sure how to make this work for all projects, but if you find out leave a comment)
9. Run your progam
You should get this:
I hope this helped you even if you didn’t have the exact specs as me.
If this isn’t working for you, leave a comment below and I’ll try to get back to you as soon as possible.